本文講述使用JSP實(shí)現(xiàn)用戶登錄,包括用戶登錄、注冊(cè)和退出功能等。
1.系統(tǒng)用例圖
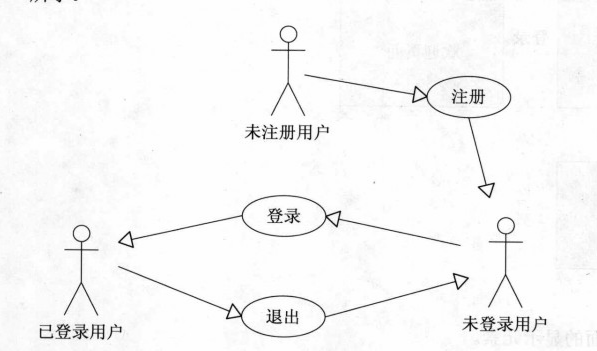
2.頁(yè)面流程圖
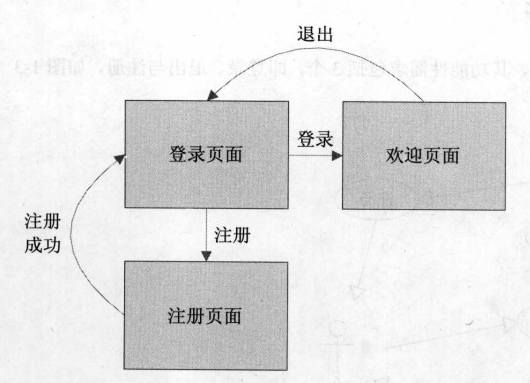
3.數(shù)據(jù)庫(kù)設(shè)計(jì)
本例使用oracle數(shù)據(jù)庫(kù)
創(chuàng)建用戶表
包括id,username,password和email,共4個(gè)字段
-- Create table
create table P_USER
(
id VARCHAR2(50) not null,
username VARCHAR2(20),
password VARCHAR2(20),
email VARCHAR2(50)
)
tablespace USERS
pctfree 10
initrans 1
maxtrans 255
storage
(
initial 64
minextents 1
maxextents unlimited
);
-- Add comments to the table
comment on table P_USER
is '用戶表';
-- Add comments to the columns
comment on column P_USER.id
is 'id';
comment on column P_USER.username
is '用戶名';
comment on column P_USER.password
is '密碼';
comment on column P_USER.email
is 'email';
4.頁(yè)面設(shè)計(jì)
4.1登錄頁(yè)面
login.jsp
%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
%>
!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
html>
head>
base href="%=basePath%>">
title>登錄頁(yè)面/title>
meta http-equiv="pragma" content="no-cache">
meta http-equiv="cache-control" content="no-cache">
meta http-equiv="expires" content="0">
meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
meta http-equiv="description" content="This is my page">
!--
link rel="stylesheet" type="text/css" href="styles.css">
-->
/head>
body>
form action="login_action.jsp" method="post">
table>
tr>
td colspan="2">登錄窗口/td>
/tr>
tr>
td>用戶名:/td>
td>input type="text" name="username" />
/td>
/tr>
tr>
td>密碼:/td>
td>input type="text" name="password" />
/td>
/tr>
tr>
td colspan="2">input type="submit" value="登錄" /> a href="register.jsp">注冊(cè)/a>
/td>
/tr>
/table>
/form>
/body>
/html>
頁(yè)面效果
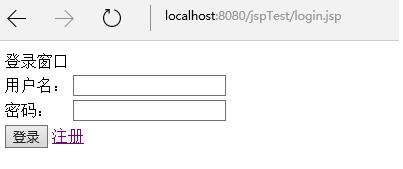
4.2登錄邏輯處理頁(yè)面
login_action.jsp
%@ page language="java" import="java.util.*" pageEncoding="utf-8"%>
%@ page import="java.sql.*" %>
%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
%>
%
String username = request.getParameter("username");
String password = request.getParameter("password");
if(username==null||"".equals(username.trim())||password==null||"".equals(password.trim())){
//out.write("用戶名或密碼不能為空!");
System.out.println("用戶名或密碼不能為空!");
response.sendRedirect("login.jsp");
return;
//request.getRequestDispatcher("login.jsp").forward(request, response);
}
boolean isValid = false;
Connection con = null;// 創(chuàng)建一個(gè)數(shù)據(jù)庫(kù)連接
PreparedStatement pre = null;// 創(chuàng)建預(yù)編譯語(yǔ)句對(duì)象,一般都是用這個(gè)而不用Statement
ResultSet result = null;// 創(chuàng)建一個(gè)結(jié)果集對(duì)象
try
{
Class.forName("oracle.jdbc.driver.OracleDriver");// 加載Oracle驅(qū)動(dòng)程序
//System.out.println("開始嘗試連接數(shù)據(jù)庫(kù)!");
String url = "jdbc:oracle:" + "thin:@127.0.0.1:1521:orcl";// 127.0.0.1是本機(jī)地址,orcl是Oracle的默認(rèn)數(shù)據(jù)庫(kù)名
String user = "scott";// 用戶名,系統(tǒng)默認(rèn)的賬戶名
String pwd = "tiger";// 你安裝時(shí)選設(shè)置的密碼
con = DriverManager.getConnection(url, user, pwd);// 獲取連接
// System.out.println("連接成功!");
String sql = "select * from p_user where username=? and password=?";// 預(yù)編譯語(yǔ)句,“?”代表參數(shù)
pre = con.prepareStatement(sql);// 實(shí)例化預(yù)編譯語(yǔ)句
pre.setString(1, username);// 設(shè)置參數(shù),前面的1表示參數(shù)的索引,而不是表中列名的索引
pre.setString(2, password);// 設(shè)置參數(shù),前面的1表示參數(shù)的索引,而不是表中列名的索引
result = pre.executeQuery();// 執(zhí)行查詢,注意括號(hào)中不需要再加參數(shù)
if (result.next()){
isValid = true;
}
}
catch (Exception e)
{
e.printStackTrace();
}
finally
{
try
{
// 逐一將上面的幾個(gè)對(duì)象關(guān)閉,因?yàn)椴魂P(guān)閉的話會(huì)影響性能、并且占用資源
// 注意關(guān)閉的順序,最后使用的最先關(guān)閉
if (result != null)
result.close();
if (pre != null)
pre.close();
if (con != null)
con.close();
//System.out.println("數(shù)據(jù)庫(kù)連接已關(guān)閉!");
}
catch (Exception e)
{
e.printStackTrace();
}
}
if(isValid){
System.out.println("登錄成功!");
session.setAttribute("username", username);
response.sendRedirect("welcome.jsp");
return;
}else{
System.out.println("登錄失??!");
response.sendRedirect("login.jsp");
return;
}
%>
使用JDBC連接數(shù)據(jù)庫(kù),如果用戶名或密碼為空時(shí),還是跳轉(zhuǎn)到登錄頁(yè)面login.jsp
如果用戶名和密碼不為空,進(jìn)行連接數(shù)據(jù)庫(kù)查詢用戶表,如果能夠查詢到記錄,表示登錄成功,將用戶信息保存到session,跳轉(zhuǎn)到歡迎頁(yè)面welcome.jsp
如果根據(jù)用戶名和密碼查詢不到記錄,表示登錄失敗,重新跳轉(zhuǎn)到登錄頁(yè)面login.jsp
4.3歡迎頁(yè)面
welcome.jsp
%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
%>
!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
html>
head>
base href="%=basePath%>">
title>My JSP 'welcom.jsp' starting page/title>
meta http-equiv="pragma" content="no-cache">
meta http-equiv="cache-control" content="no-cache">
meta http-equiv="expires" content="0">
meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
meta http-equiv="description" content="This is my page">
!--
link rel="stylesheet" type="text/css" href="styles.css">
-->
/head>
body>
table>
tr>
td>img src="images/logo4.png" />
/td>
td>img src="images/logo2.png" height="90" />
/td>
/tr>
tr>
td colspan="2">hr />
/td>
/tr>
tr>
td>
table>
tr>
td>a>Main/a>
/td>
/tr>
tr>
td>a>Menu1/a>
/td>
/tr>
tr>
td>a>Menu2/a>
/td>
/tr>
tr>
td>a>Menu3/a>
/td>
/tr>
tr>
td>a>Menu4/a>
/td>
/tr>
tr>
td>a>Menu5/a>
/td>
/tr>
tr>
td>a>Menu6/a>
/td>
/tr>
tr>
td>a>Menu7/a>
/td>
/tr>
tr>
td>a>Menu8/a>
/td>
/tr>
/table>/td>
td>
form action="loginout.jsp" method="post">
table>
tr>
td colspan="2">登錄成功!/td>
/tr>
tr>
td>歡迎你,/td>
td>${username }/td>
/tr>
tr>
td colspan="2">input type="submit" value="退出" />/td>
/tr>
/table>
/form>/td>
/tr>
/table>
/body>
/html>
使用EL表達(dá)式展示用戶信息
效果
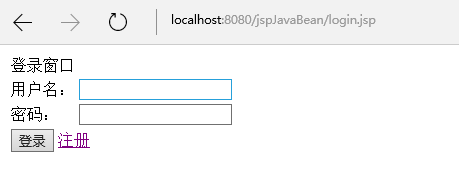
4.4歡迎頁(yè)退出邏輯處理頁(yè)面
loginout.jsp
%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
%>
!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
html>
head>
base href="%=basePath%>">
title>My JSP 'loginout.jsp' starting page/title>
meta http-equiv="pragma" content="no-cache">
meta http-equiv="cache-control" content="no-cache">
meta http-equiv="expires" content="0">
meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
meta http-equiv="description" content="This is my page">
!--
link rel="stylesheet" type="text/css" href="styles.css">
-->
/head>
body>
%
session.removeAttribute("username");
response.sendRedirect("login.jsp");
%>
/body>
/html>
將session的用戶信息移除,跳轉(zhuǎn)到登錄頁(yè)面login.jsp
4.5注冊(cè)頁(yè)面
register.jsp
%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
%>
!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
html>
head>
base href="%=basePath%>">
title>注冊(cè)頁(yè)面/title>
meta http-equiv="pragma" content="no-cache">
meta http-equiv="cache-control" content="no-cache">
meta http-equiv="expires" content="0">
meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
meta http-equiv="description" content="This is my page">
!--
link rel="stylesheet" type="text/css" href="styles.css">
-->
/head>
body>
form action="register_action.jsp" method="post">
table>
tr>
td colspan="2">注冊(cè)窗口/td>
/tr>
tr>
td>用戶名:/td>
td>input type="text" name="username" />/td>
/tr>
tr>
td>密碼:/td>
td>input type="text" name="password1" />/td>
/tr>
tr>
td>確認(rèn)密碼:/td>
td>input type="text" name="password2" />/td>
/tr>
tr>
td>email:/td>
td>input type="text" name="email" />/td>
/tr>
tr>
td colspan="2">input type="submit" value="注冊(cè)" /> a href="login.jsp">返回/a>/td>
/tr>
/table>
/form>
/body>
/html>
當(dāng)在登錄頁(yè)面點(diǎn)擊“注冊(cè)“時(shí)打開用戶注冊(cè)頁(yè)面
效果

4.6注冊(cè)邏輯處理頁(yè)面
register_action.jsp
%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
%@ page import="java.sql.*" %>
%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
%>
%
String username = request.getParameter("username");
String password1 = request.getParameter("password1");
String password2 = request.getParameter("password2");
String email = request.getParameter("email");
if(username==null||"".equals(username.trim())||password1==null||"".equals(password1.trim())||password2==null||"".equals(password2.trim())||!password1.equals(password2)){
//out.write("用戶名或密碼不能為空!");
System.out.println("用戶名或密碼不能為空!");
response.sendRedirect("register.jsp");
return;
//request.getRequestDispatcher("login.jsp").forward(request, response);
}
boolean isValid = false;
Connection con = null;// 創(chuàng)建一個(gè)數(shù)據(jù)庫(kù)連接
PreparedStatement pre = null;// 創(chuàng)建預(yù)編譯語(yǔ)句對(duì)象,一般都是用這個(gè)而不用Statement
ResultSet result = null;// 創(chuàng)建一個(gè)結(jié)果集對(duì)象
try
{
Class.forName("oracle.jdbc.driver.OracleDriver");// 加載Oracle驅(qū)動(dòng)程序
//System.out.println("開始嘗試連接數(shù)據(jù)庫(kù)!");
String url = "jdbc:oracle:" + "thin:@127.0.0.1:1521:orcl";// 127.0.0.1是本機(jī)地址,orcl是Oracle的默認(rèn)數(shù)據(jù)庫(kù)名
String user = "scott";// 用戶名,系統(tǒng)默認(rèn)的賬戶名
String pwd = "tiger";// 你安裝時(shí)選設(shè)置的密碼
con = DriverManager.getConnection(url, user, pwd);// 獲取連接
//System.out.println("連接成功!");
String sql = "select * from p_user where username=?";// 預(yù)編譯語(yǔ)句,“?”代表參數(shù)
pre = con.prepareStatement(sql);// 實(shí)例化預(yù)編譯語(yǔ)句
pre.setString(1, username);// 設(shè)置參數(shù),前面的1表示參數(shù)的索引,而不是表中列名的索引
result = pre.executeQuery();// 執(zhí)行查詢,注意括號(hào)中不需要再加參數(shù)
if (!result.next()){
sql = "insert into p_user(id,username,password,email) values(?,?,?,?)";// 預(yù)編譯語(yǔ)句,“?”代表參數(shù)
pre = con.prepareStatement(sql);// 實(shí)例化預(yù)編譯語(yǔ)句
pre.setString(1, System.currentTimeMillis()+"");// 設(shè)置參數(shù),前面的1表示參數(shù)的索引,而不是表中列名的索引
pre.setString(2, username);// 設(shè)置參數(shù),前面的1表示參數(shù)的索引,而不是表中列名的索引
pre.setString(3, password1);// 設(shè)置參數(shù),前面的1表示參數(shù)的索引,而不是表中列名的索引
pre.setString(4, email);// 設(shè)置參數(shù),前面的1表示參數(shù)的索引,而不是表中列名的索引
pre.executeUpdate();// 執(zhí)行
isValid = true;
}
}
catch (Exception e)
{
e.printStackTrace();
}
finally
{
try
{
// 逐一將上面的幾個(gè)對(duì)象關(guān)閉,因?yàn)椴魂P(guān)閉的話會(huì)影響性能、并且占用資源
// 注意關(guān)閉的順序,最后使用的最先關(guān)閉
if (result != null)
result.close();
if (pre != null)
pre.close();
if (con != null)
con.close();
//System.out.println("數(shù)據(jù)庫(kù)連接已關(guān)閉!");
}
catch (Exception e)
{
e.printStackTrace();
}
}
if(isValid){
System.out.println("注冊(cè)成功,請(qǐng)登錄!");
response.sendRedirect("login.jsp");
return;
}else{
System.out.println("用戶名已存在!");
response.sendRedirect("register.jsp");
return;
}
%>
首先判斷用戶名和密碼是否為空,以及密碼和確認(rèn)密碼是否一致,如果上述條件不成立時(shí),返回到注冊(cè)頁(yè)面register.jsp
如果上述條件成立,就根據(jù)用戶名到數(shù)據(jù)庫(kù)查詢,如果能夠查詢到記錄,說(shuō)明用戶名已經(jīng)存在,返回到注冊(cè)頁(yè)面register.jsp
如果查詢不到記錄,說(shuō)明此用戶名可用來(lái)進(jìn)行注冊(cè),使用JDBC向用戶表 插入1條記錄;之后跳轉(zhuǎn)到登錄頁(yè)面login.jsp
5.總結(jié)
本例使用JSP實(shí)現(xiàn)用戶登錄,編寫過(guò)程中,主要遇到了2個(gè)小問(wèn)題。
5.1查詢之后,判斷記錄是否存在,需要使用 if (!result.next()),而不是通常查詢中使用的while循環(huán),這一點(diǎn)需要注意,特別是在處理注冊(cè)時(shí)。
5.2關(guān)于JSP頁(yè)面的編譯報(bào)錯(cuò)問(wèn)題。
當(dāng)在JSP小腳本中中使用return時(shí)要慎重,很可能會(huì)出現(xiàn)編譯錯(cuò)誤。
處理方法是,JSP主頁(yè)面只使用JSP小腳本,保證return之后沒有還需要編譯的內(nèi)容即可。
以上即為使用JSP實(shí)現(xiàn)用戶登錄的簡(jiǎn)單介紹,希望對(duì)大家的學(xué)習(xí)有所幫助。
您可能感興趣的文章:- jsp+dao+bean+servlet(MVC模式)實(shí)現(xiàn)簡(jiǎn)單用戶登錄和注冊(cè)頁(yè)面
- 使用JSP實(shí)現(xiàn)簡(jiǎn)單的用戶登錄注冊(cè)頁(yè)面示例代碼解析
- jsp實(shí)現(xiàn)用戶自動(dòng)登錄功能
- jsp實(shí)現(xiàn)簡(jiǎn)單用戶7天內(nèi)免登錄
- servlet+jsp實(shí)現(xiàn)過(guò)濾器 防止用戶未登錄訪問(wèn)
- JSP Spring防止用戶重復(fù)登錄的實(shí)現(xiàn)方法
- JavaWeb實(shí)現(xiàn)用戶登錄注冊(cè)功能實(shí)例代碼(基于Servlet+JSP+JavaBean模式)
- jsp基于XML實(shí)現(xiàn)用戶登錄與注冊(cè)的實(shí)例解析(附源碼)
- JSP實(shí)現(xiàn)簡(jiǎn)單的用戶登錄并顯示出用戶信息的方法
- 在jsp中用bean和servlet聯(lián)合實(shí)現(xiàn)用戶注冊(cè)、登錄
- 關(guān)于JSP用戶登錄連接數(shù)據(jù)庫(kù)詳情